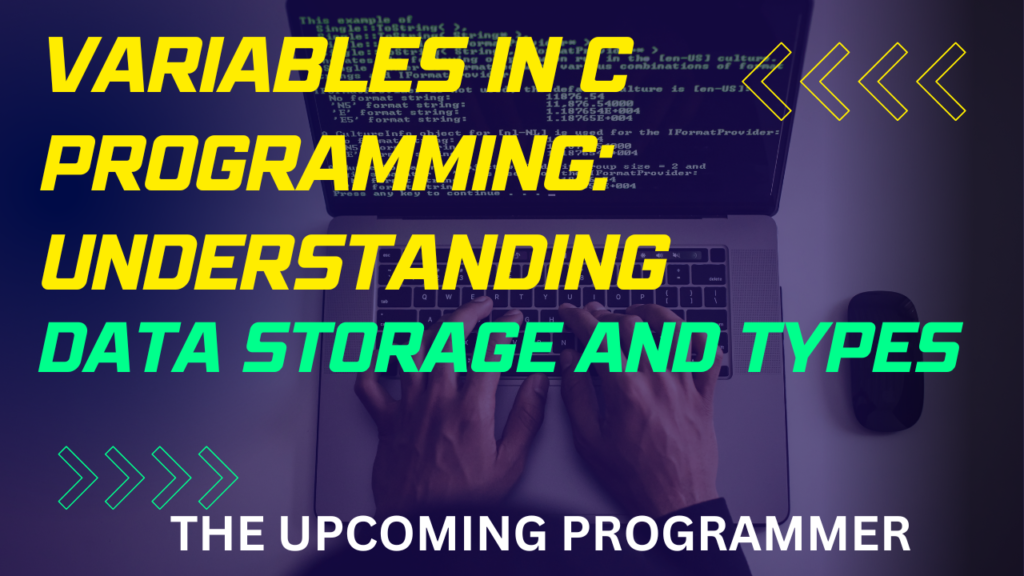
What are variables in C?
Variables are an essential part of programming in C, as they allow us to store and manipulate data in our programs. By assigning a value to a variable, we can store that value in memory and then refer to it using the variable’s name. It’s important to declare variables with their data type before using them in a program, as this tells the compiler how much memory to allocate for the variable and how to interpret the data stored there. C supports several different data types for variables, including integers, floating-point numbers, characters, and strings.
What is variable and its type?
Variable names should be chosen carefully to reflect the purpose of the variable and to make the program more readable. While variable names can contain letters, digits, and underscores, they must begin with a letter or underscore and cannot begin with a digit. Additionally, variable names in C are case sensitive, so “myVar” and “myvar” would be considered two different variables.
One of the great advantages of using variables in C is that we can change their values during the course of a program. By assigning a new value to a variable, we can update the data stored in memory and use it in calculations and other operations. This flexibility is crucial in creating programs that can perform complex tasks and respond to changing conditions. Overall, variables are a fundamental concept in C programming that every developer should understand.
#include <stdio.h> int main() { // Declare and initialize variables int num1 = 10; int num2 = 20; int sum; // Calculate sum sum = num1 + num2; // Print result printf("The sum of %d and %d is %d.\n", num1, num2, sum); // Declare and initialize more variables float pi = 3.14159; char letter = 'A'; double bigNumber = 1234567890123456.789; // Print the values of the variables printf("The value of pi is %f.\n", pi); printf("The letter is %c.\n", letter); printf("The big number is %f.\n", bigNumber); return 0; }
Output
The sum of 10 and 20 is 30.
The value of pi is 3.141590.
The letter is A.
The big number is 1234567890123456.750000.