Table of Contents
What is Structure ?
Structures, also known as structs, are a method of gathering various related variables into a single unit. The structure refers to each variable as a member.. A structure can hold numerous data types like integers, decimals, or characters, unlike arrays. This makes structures useful for organizing and storing related data. Structures allow you to define your own custom data types to represent real-world objects or concepts in your program. Structures streamline code by grouping variables, allowing for easier access and improved organization.
Advantages of Using Structures in C Programming for Data Management
- Structures facilitate organization by allowing you to group related variables into a single unit, making your code more organized, readable, and comprehensible.
- Structures enable you to manage complex data by combining variables of different data types into a single unit, which simplifies the process of manipulating and working with data.
- Structures allow you to create custom data types that represent real-world objects or concepts, making your code more intuitive and easy to work with.
- You can easily pass structures as arguments to functions, which enables you to work with complex data sets more easily and efficiently in your programs.
- Using structures can help you manage memory more efficiently, as you can allocate and deallocate memory for the entire structure at once.
- In summary, structures are a powerful tool in C programming that simplifies the process of organizing and manipulating data, leading to more organized, maintainable, and scalable code.
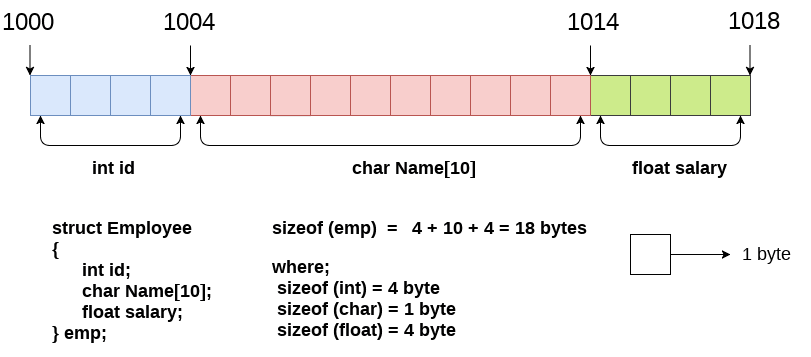
The syntax of a structure in C programming is as follows:
struct structureName { dataType member1; dataType member2; // add as many members as needed }; // also you can declare it as struct structureName variableName;
This creates a new variable of the structure type structureName
. To access the members of a structure variable, you use the dot operator (.) followed by the member name, like this:
variableName.member1 = value; variableName.member2 = value;
Here are the main points to create a new structure in C programming:
- Use the “struct” keyword to define a new structure.
- You should give the structure a name, which will be referred to as “structureName”.
- Assign a data type to each member variable in the structure using the “dataType” keyword.
- Define the member variables themselves and name them appropriately, such as “member1” and “member2”.
- Each member variable can be of any data type, providing flexibility in how you organize your data.
Example of Structures in C Programming
#include <stdio.h> // Define the structure struct person { char name[50]; int age; float height; }; int main() { // Declare and initialize a structure variable struct person p1 = {"John", 30, 1.75}; // Access the members of the structure using the dot operator printf("Name: %s\n", p1.name); printf("Age: %d\n", p1.age); printf("Height: %.2f\n", p1.height); return 0; }
- Code defines a structure named person with three members.
- Members are name, age, and height.
- In main, a person variable named p1 is declared and initialized.
- Dot operator used to access members of p1 variable.
- printf statements demonstrate accessing members.
- Output displays values of members.
Drawbacks of Structures in C Programming
Structures in C programming come with certain drawbacks that must be taken into account while designing and implementing programs. These drawbacks can be listed out as follows:
Firstly, structures can lead to memory inefficiency as they require a fixed amount of memory to be allocated for each member, even if some members do not require the full amount of memory assigned to them. This results in wastage of memory resources, which can be detrimental to program performance and efficiency.
Secondly, manipulating and passing structures in functions can be a challenging task. Unlike single members, entire structures require passing around a pointer to the structure, making the process more complicated and time-consuming.
Thirdly, using structures improperly can lead to difficult-to-debug errors such as memory overwrites or uninitialized values. Proper initialization and management of structure members are crucial to avoid such errors, which can be troublesome to detect and fix.
In conclusion, while structures can be advantageous in organizing related data in a program, it is essential to consider their drawbacks and handle them properly during the program design and implementation phase.