In simple and easy-to-understand language, a pointer in C programming is a variable that stores the location in memory of another variable. It allows developers to handle memory in a more efficient manner, work with data structures more easily, and pass variables to functions by reference.
Pointers also enable dynamic memory allocation and deallocation at runtime, which is a significant advantage in programming. Programmers commonly use pointers to navigate through arrays and linked lists, which makes them an essential component of C programming.
To create a pointer in C, you use the *
symbol and indicate the data type of the variable that the pointer points to. You can set the pointer to hold the memory address of another variable by using the &
operator.
By understanding how pointers operate, you can create more optimized and effective code in C.
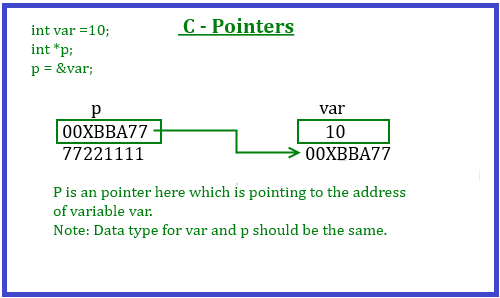
In contrast to static allocation, pointers in C programming enable dynamic memory allocation and deallocation at runtime and hold the memory address of another variable. They allow for the efficient sending of arguments by reference to functions, which can be more effective than providing parameters by value. Programmers frequently use pointers to effectively handle data structures such as arrays and linked lists.
It is possible to efficiently browse between arrays and structures using pointer arithmetic. Dereferencing the pointer (using the * operator) will allow you to access the value kept in the memory address that it points to. To avoid undefined behavior, you must first assign a value to uninitialized pointers before utilizing them.
To prevent mistakes and possible memory leaks, it’s crucial to utilize pointers properly. Using an erroneous
Syntax of Pointer in C programming
data_type *pointer_name; int *ptr; pointer_name = &variable_name; ptr = # data_type *pointer_name = &variable_name; int *ptr = #
Example Of Pointer in C programming
#include <stdio.h> int main() { int num = 10; // declare and initialize an integer variable num int *ptr; // declare a pointer to an integer ptr = # // assign the address of num to ptr printf("Address of num: %p\n", &num); printf("Value of ptr: %p\n", ptr); // print the value of ptr (the address of num) printf("Value of num: %d\n", *ptr); // print the value stored at the address pointed to by ptr *ptr = 20; // change the value of num through the pointer printf("Value of num: %d\n", num); // print the new value of num return 0; }
To illustrate the use of pointers in C programming, we begin by declaring an integer variable num
with the value 10
. We then create a pointer variable ptr
that can hold the memory address of an integer.
We use the &
operator to assign the memory address of num
to ptr
. We print the address of num
, the value stored in ptr
(which is the address of num
), and the value stored at the memory address pointed to by ptr
.
We demonstrate the flexibility of pointers by changing the value of num
using the pointer. We do this by assigning the value 20
to the memory location pointed to by ptr
. Finally, we print the updated value of num
to confirm that the pointer has successfully modified its value.
Output
Address of num: 0x7ffd3a21d7a4
Value of ptr: 0x7ffd3a21d7a4
Value of num: 10
Value of num: 20
Frequently Asked Questions on Pointers in C Programming
In C programming, a pointer is a variable that holds the memory address of another variable. Pointers are used to manipulate and access memory directly, allowing for more efficient memory usage and enabling the creation of dynamic data structures like linked lists, trees, and graphs.
A pointer in C programming is a variable that stores the memory address of another variable. Pointers are used to manipulate data stored in memory and to dynamically allocate and deallocate memory.
There are three types of pointers in C programming:
Null Pointer:
Void Pointer:
Function Pointer:
In C programming, a null pointer is a pointer that does not point to any memory location. It is a special value that is used to indicate that the pointer is not currently pointing to a valid object or function. The null pointer is represented in C by the constant value “NULL”, which is defined as a zero-valued expression.
A void pointer in C is a special type of pointer that can point to a memory location of any data type. It is also known as a generic pointer because it can be used to point to any data type, including integers, characters, floating-point numbers, arrays, structures, and functions.
The void pointer is declared using the “void” keyword,
In C programming, the size of a pointer depends on the underlying architecture of the system being used.
- On a 32-bit system, the size of a pointer is typically 4 bytes,
- on a 64-bit system, it is usually 8 bytes.
In C programming, pointers are declared using the following syntax:
data_type *pointer_variable_name;
int *ptr;