Table of Contents
Definition
A while loop is a programming concept that repeats a set of instructions over and over again until a certain condition is no longer true. This allows the loop to keep going as long as the condition is true, and stops when it becomes false.
Have you ever found yourself in a situation where you needed to repeat a task multiple times but didn’t know how many times it would need to be done? In such a scenario, while loops can be very helpful. These loops allow you to keep performing an action until a specific condition is met. While loops can be incredibly helpful when dealing with large datasets or performing an action on several items in a list.
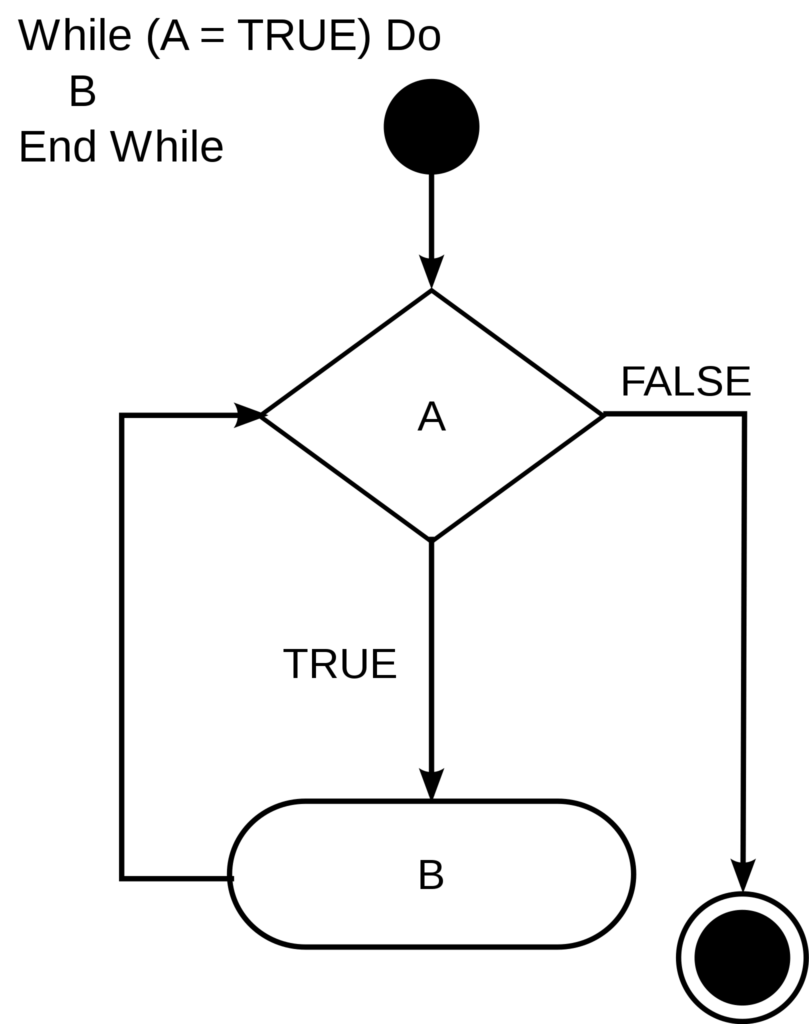
The while loop is an essential concept to understanding programming. It allows for the repetition of a task until a certain condition is met. With the use of a while loop, the program can continue running until a certain break point is reached. This can be particularly useful in situations where the exact number of iterations needed is unknown.:
The syntax of a while loop in most programming languages is as follows:
while (condition) { // code to be executed while the condition is true }
An Example of a while loop in C programming:
#include <stdio.h> int main() { int i = 1; while (i <= 10) { printf("%d ", i); i++; } return 0; }
in this example, the while loop will execute as long as the variable i
is less than or equal to 10. Inside the loop, the value of i
is printed using the printf
function, and then i
is incremented by 1 using the i++
shorthand for i = i + 1
. This process continues until i
becomes 11, at which point the loop stops and the program ends.
Output
1 2 3 4 5 6 7 8 9 10
Recent Posts
- How to Get Started with Deepseek: A Step-by-Step Guide for Beginners
- The Future of Deepseek: Trends and Predictions for 2024 and Beyond
- Top 10 Use Cases of Deepseek for Businesses and Individuals
- Deepseek vs. Competitors: Why It Stands Out in the Market in 2025
- How Deepseek is Revolutionizing [Industry/Field]: Key Insights in 2025