What is the goto statement in C?
The goto
statement in C programming allows you to jump to a specific labeled statement in your code, which can change the order in which your program runs. However, using goto
statements can make your code difficult to read and debug, so it is generally recommended to use other control flow statements like if
, while
, for
, and switch
instead. These statements make your code easier to understand and maintain.
Syntax of goto Statement in C Programming:
goto label; /* code... */ label: /* statement */
When the goto
statement is executed, the program jumps to the labeled statement, which is defined elsewhere in the code. The label is identified by a unique name followed by a colon (:
) character. Once the labeled statement is executed, the program continues to execute from that point on.
The use of goto
statements can make your code more difficult to read and maintain, and can potentially lead to hard-to-find bugs or infinite loops. As a result, it is generally recommended to avoid using goto
statements in modern programming languages, and to use structured control flow statements like if
, while
, for
, and switch
instead.
.
What is goto in flowchart?
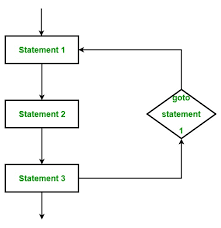
Example of go to Statements
#include <stdio.h> int main() { int num = 1; loop: // this is the labeled statement printf("%d\n", num); num++; if (num <= 10) { goto loop; // this statement jumps back to the labeled statement } return 0; }
- We construct a loop that outputs the digits 1 through 10 using the goto expression.
Loop is a labelled statement that we define. The loop’s starting point is indicated by this labelled statement. - The goto statement is used to return to the labelled statement loop whenever we wish to restart the loop.
We display the value of num, which starts at 1, within the loop.
We increase num by 1 after printing its value.
Whether num is less than or equal to 10 is determined. If so, we return to the labelled statement loop and execute the loop once more. If not, the programme ends and we break out of the loop.
Frequently Asked Questions
The goto
statement in C is a control flow statement that allows the program to jump to a labeled statement elsewhere in the code. It is often considered a controversial feature of the language because it can make code harder to read and understand, and it can lead to problems such as infinite loops or unintended jumps in the code.
The goto
statement in C has the following syntax:
goto label;
label:
“Goto“, on the other hand, specifically refers to the goto
statement in C, which allows the program to transfer control flow to a labeled statement elsewhere in the code. The goto
statement is often considered a controversial feature of the language because it can make code harder to read and understand.
“Jump” is a more general term that refers to any type of control flow transfer, whether it be through a function call, a loop, or a conditional statement. In this sense, “jump” can be seen as a more abstract concept that encompasses all types of control flow transfers.
- The
goto
statement in C is a control flow statement that can transfer program execution to a labeled statement elsewhere in the code. - The
goto
statement can be useful in certain situations, such as error handling or breaking out of nested loops. - However, the use of
goto
is generally discouraged in modern programming practice, as it can make code harder to read and maintain. - Using structured control flow statements such as
if
,while
,for
, andswitch
is preferred over usinggoto
. - In general,
goto
should be used sparingly and only when there is no better alternative.
#include <stdio.h>
int main() {
int x = 0;
start:
printf(“The value of x is %d\n”, x);
x++;
if (x < 10) {
goto start;
}
return 0;
}
- Understanding Identifiers in C++ Programming: An Introduction to Naming Variables, Functions, and Labels
- 1What is C++ Programming? A Historical Overview and Introduction to the Fundamentals and Features
- 2Smart Shopping: How to Find the Best Laptops Under 60,000 Rupees
- 3Chat GPT Explained: Everything You Need to Know About OpenAI’s Language Model
- 4Understanding Union in C Programming: Exploring Data Type Aliasing and Effective Usage
- 5Understanding the Syntax and Functionality of Structures in C Programming